Description
Mark a method as an interactive command.
Mark a public method with the Command attribute.
The method HelloX
requires a string and returns a string. The [Command]
attribute marks it as an interactive command to Minibuffer by the name 'hello-x', which can be executed by typing M-x hello-x. Minibuffer will then prompt the user String name: . Suppose the user responds Mary. The method will return a string "Hello, Mary", and Minibuffer will show this as a message Hello, Mary.
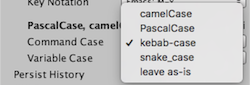
- Note
- The naming convention for commands may be set to PascalCase, camelCase, and kebab-case, or as-is. So the
HelloX
command name would be "HelloX", "helloX", or "hello-x" respectively. See CanonizeCommand for more details.
Registering with Minibuffer
Classes do have to register with Minibuffer.
Specify the command name
Type M-x hi to run the following command.
Provide a description
Explain what a command does in its description that is used in various help commands like M-x describe-command and M-x describe-bindings.
See its description by typing M-x describe-command hi-there.
hi-there is not bound to any keys. Description: "Say hello to X." It is defined in class ExampleCommands. String HelloW(String str)
Set a key binding
Bind a key to the command. Run the command M-x hello-z or by typing C-h w.
See listing of all builtin Commands, or type C-h c all.
Prompts
The default prompt for each command argument is "<type> <argument-name>: ". This can be changed by adding a Prompt attribute.
See the Prompt class for more details.
Special Return Types
Minibuffer commands that return a String
or IEnumerator
are handled differently for convenience.
String Return Type
A command that returns a String
is output as a message.
It is equivalent to the following code.
IEnumerator Return Type
A command that returns an IEnumerator
is run as a coroutine.
It is equivalent to the following code.
Public Member Functions | |
Command () | |
The command name will be derived from the method's name. More... | |
Command (string name) | |
Command with given name. | |
Public Attributes | |
string | name |
Name of the command. More... | |
string | description |
Command description or null. More... | |
string | briefDescription |
The brief description will be generated from the first sentence of the description if available; otherwise it's null. More... | |
string | keyBinding |
Bind a key sequence to this command. More... | |
string[] | keyBindings |
Bind multiple key sequences to this command. More... | |
string | keymap |
The keymap to place any key bindings in. More... | |
string | group |
Place command in given group. More... | |
Constructor & Destructor Documentation
Command | ( | ) |
The command name will be derived from the method's name.
Member Data Documentation
string name |
Name of the command.
If not given, will use the method's name.
string description |
Command description or null.
string briefDescription |
The brief description will be generated from the first sentence of the description if available; otherwise it's null.
string keyBinding |
Bind a key sequence to this command.
string [] keyBindings |
Bind multiple key sequences to this command.
string keymap |
The keymap to place any key bindings in.
string group |
Place command in given group.
By default it will go into the group of its class.